Godot: walking and turn around
This is an example of how I implemented (on Godot 3.4.2 stable) a walking and turning around system for a
protagonist character.
- extends KinematicBody
- export var velocity = 10
- onready var camera = $"../pointOfView/Camera"
- onready var navigation = $"../navigation"
- var spacestate = PhysicsDirectSpaceState
- var path = []
- var pathidx = 0
- var animstate = 0 # 0 => idle ; 1 => walking
- func _ready():
- spacestate = get_world().direct_space_state
- func _input(event):
- var mouseevent = event as InputEventMouseButton
- if not mouseevent:
- return
- if mouseevent.pressed and mouseevent.button_index == 1:
- var from = camera.project_ray_origin(mouseevent.position)
- var to = from + camera.project_ray_normal(mouseevent.position) * 200
- var res = spacestate.intersect_ray(from, to)
- if not res.empty():
- path = navigation.get_simple_path(global_transform.origin, res.position)
- # Reset the index
- pathidx = 0
- # Begin of the walking
- $"protagonist/AnimationPlayer".play("walk", 0.5)
- animstate = 1 # walk
- func _physics_process(delta):
- if pathidx < path.size():
- var direction = path[pathidx] - global_transform.origin
- if direction.length() < 1:
- pathidx += 1
- else:
- move_and_slide(direction.normalized() * velocity)
- func _process(delta):
- # The character turns around
- if pathidx < path.size():
- var newT = global_transform.looking_at(path[pathidx], Vector3.UP)
- global_transform = global_transform.interpolate_with(newT, 1.0 * delta)
- # Finish the walking when the character doesn't have
- # any path to follow.
- if animstate == 1 and pathidx < path.size():
- var distance = global_transform.origin.distance_to(path[path.size()-1])
- if distance < 1.5:
- animstate = 0
- $"protagonist/AnimationPlayer".play("idle", 0.5)
This is a KinematicBody with a "protagonist" child. The protagonist follows a "path", and the end of the path is the destination point. With
looking_at()
and interpolate_with()
the protagonist
will progressively turn around through the destination point. It's also featuring the start and the end of a
"walk".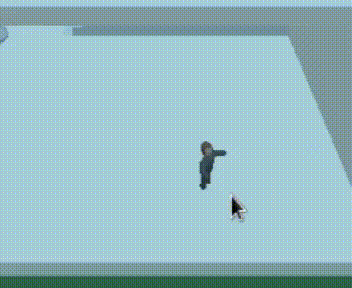
2022
Feb, 21
Feb, 21